Psionic programming, part 2
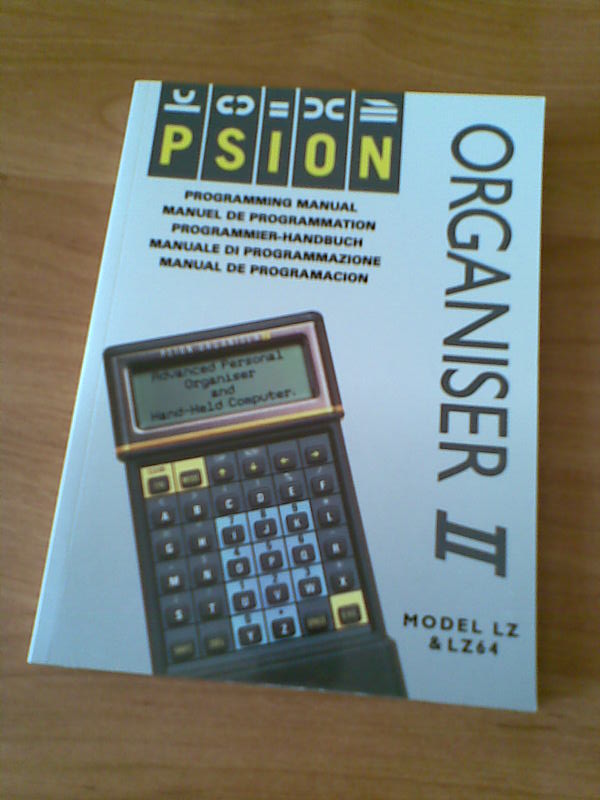
First steps
So you want to write programs. Ok, here are the first steps you should follow:
1.
Sit down, take a deep breath and think, what kind of software you'd like to create. Should it be a game? a finance manager? a pack of useful scripts?
2.
What is the destination platform? In other words, on which model will you run your program? Organiser II? Series 5mx? Or maybe you're about to make a cross-platform application?
3.
Now that you've completed point 1 and 2, choose the best tool. OPL seems to be the best choice for most applications, at least for beginners. It will give you a wide variety of useful functions, covering graphics/animation, sound, GUI and integration with built-in software. On the other side, assembler will give you freedom, joy of hacking and great performance - of course if you're already familiar with assembly programming. However, it's pointless to write a home expense tracking suite with an extensive GUI in assembly language.
If you're planning a cross-psion application, running on the Series 7 as well as on Series 3, choose OPL. This will save you a lot of work you would spend porting your program to other platforms.
If your program will run only on EPOC machines, you may want to choose some more "mainstream" language, like Python, Perl, Java or Lua - especially if you have some previous experience. In this case, come back to the first part and read about the limitations. Having chosen one of the abovementioned, remember that you need to supply the users (if you're willing to publish your work) with the runtime libraries. It's really straightforward with Lua: there are separate .SIS packages available and all you need to do is to put them together with your application. Java needs JVM, the virtual machine available with the SDK (see part 1). Perl and Python require the interpreter to be installed.
4.
Ok, you have chosen your tool? It's time to learn to use it. For OPL and SIBO/EPOC machines, read "Programming Psion Computers" - the Bible of Psion programming. Organiser programming tutorials may be found on the Organiser II homepage (see part 1).
If you have chosen assembler, you not only need to learn the mnemonics (assembly commands) for your platform, but also should become familiar with your computer's internals - read "Programming Psion computers" or the manuals on Org II homepage.
For those who want to write in OPL, especially for future game developers, there's an excellent programming tutorial in EPOC Entertainer, covering everything needed to write your own application - the tutorial takes you through the steps of game development. You can also look for some OPL tutorials for Symbian, e.g. this one.
Perl, Python, Lua and Java tutorials were mentioned in the first part.
5.
Code, code, code. Practice makes perfect.
Programming graphics on Portfolio, part 2
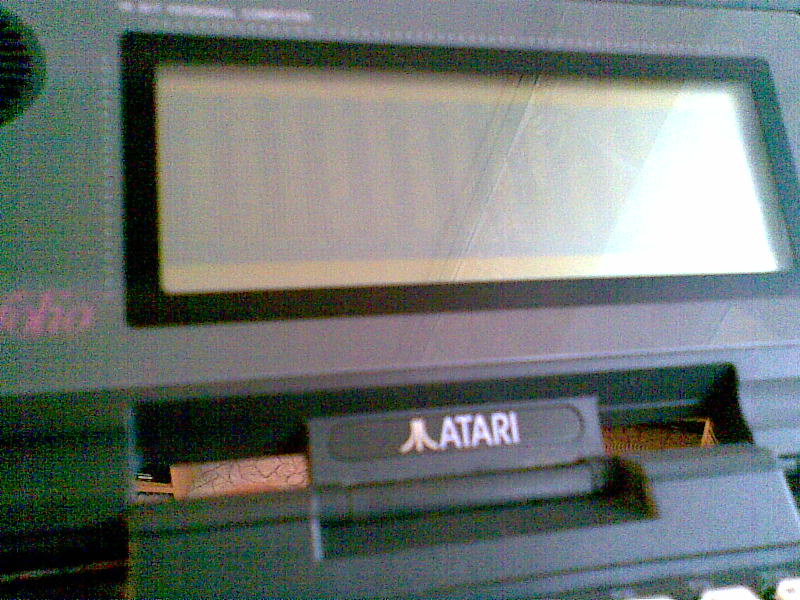
Portfolio's display is controlled by a Hitachi HD61830 chip. As I previously said, this makes it incompatible with the PC and more difficult to program. However, the only way to use dynamic graphics in your programs is to access the controller directly.
If you'd like to update the graphics in real time (e.g. in a game), the best idea would be to redraw the screen at once, so that you don't need to bother drawing each pixel separately. It is possible thanks to the routine by Milan and Martin Hrdlička:
refresh: cld push ax push cx push dx push bx push si push di push ds mov si, 0 mov ax, 0b000h mov ds, ax mov di, 64 ref2: mov cx, 30 mov bx, si mov al, 0ah mov dx, 8011h cli out dx, al mov al, bl dec dx out dx, al sti mov al, 0bh inc dx cli out dx, al dec dx mov al, bh and al, 7 out dx, al sti ref1: lodsb ror al, 1 mov ah, al and ah, 136 ror al, 1 ror al, 1 mov bl, al and bl, 68 or ah, bl ror al, 1 ror al, 1 mov bl, al and bl, 34 or ah, bl ror al, 1 ror al, 1 and al, 17 or al, ah mov ah, al inc dx mov al, 0ch cli out dx, al mov al, ah dec dx out dx, al sti loop ref1 dec di jnz ref2 pop ds pop di pop si pop bx pop dx pop cx pop ax ret
Now, all you need to do is to update the graphics at B000:0000 each time before refreshing the screen. One pixel is one bit, so there are 64 lines by 240/8=30 bytes. That makes 1920 bytes and the actual position of a pixel in memory may be calculated using this formula:
byte = y * 30 + x / 8
bit = x mod 8
so, for example to draw the point at x=50, y=17 (0,0 is the top-left corner, 239,63 is the bottom-right) you need to set the bit number 2 at B000:0204 (that is 516 decimal). Obviously, clearing the bit clears the pixel.
More tips on programming graphics:
- Portfolio News #4
- An article by Martin Řehák (in Czech)
Psionic programming, part 1
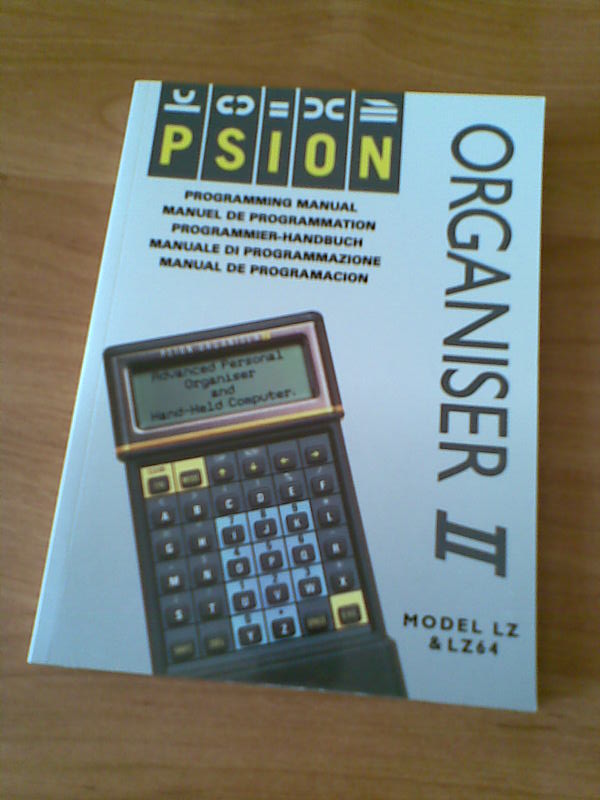
From Organiser to Series 5: Programming tools
It's not so far from the truth, that Psion Company has set the standards for many modern portable computers. Their sophisticated, sometimes revolutionary solutions pioneered the development of palmtops. Unfortunately, one of the great features of Psions not followed by today's palmtop market was the OPL. The idea of a built-in programming language wasn't new in those times - all home computers had BASIC interpreter on board - but OPL joined the ease of BASIC with the structural syntax and was well integrated with Psion's built-in facilities. And it was backwards compatible, as long as no system-specific functions were used. This means that you should be able to run your software written for the Organiser on a Symbian smartphone with just a few modifications! [1]
Apart from standalone programs, you can extend your system with OPL. The Calculator application on Series 3 Psions can perform calculations using built-in and user's own OPL functions. Also macros for Macro5 are written in OPL. On the Series 5 OPL programs can be extended by OPX modules written in C++. However, although OPL is the simplest way to write programs for Psions, it's not the only one.
On the opposite of OPL there are assemblers, allowing you to access directly machine's "guts". Since different models have different processors, it isn't possible to run your program designed for an Organiser on Series 3. Even Series 3 and 5 are incompatible.
Psion Organiser II models have an 8-bit HD6303 processor. You can program it using PCMAC. It's a cross-assembler, i.e. it's run on a PC and the generated machine code must be transferred to the Organiser.
All Series 3 models and the Siena have a 16-bit NEC V30 - which in fact is a low-consumption 8086 clone (although in different models it's clocked at different speeds). This means that you may use an x86 assembler, compile the code on a PC and it will be ready to run on the Psion. There are tons of commercial (TASM, MASM) as well as free- or shareware (A86, New Basic Assembler) assemblers. If you're willing to do the work on your Psion, there's even one that runs natively on your machine - S3A.
Series 5 models are powered by a 32-bit ARM710T. I know of two assemblers for them, both running on the Psion. The first is French Assembleur (unfortunately, the instruction manual is also in French) and the second is called simply ASM. I also read about "GB Assembler Studio" by Nicklas Larsson, but it seems to have disappeared from the Web.
Something else? Sure. Series 3 computers can be programmed in C. You'll find some good resources on Gareth and Jane Saunders' website. They have also some goodies for those who would like to write programs in C++ for their Series 5.
Of course that's not all. There are many more, especially for Psion Series 5. Among them are two Titans: Perl 5.6.1 and Python 2.2.1. (There's also Python 2.1 port, Epocpython). To me, the greatest drawback of these ports is the lack of Psion API (or I haven't found it). So they may be good for prototyping (in a limited manner, as Python 2.2 is an ancient version, eons before 2.7, not to mention 3k) or running some useful scripts (keep in mind, that they'll execute really slow), but nothing more. No real Psion applications written in Python/Perl. What a pity.
For those who want to program in a well-known, multi-platform and powerful language, there's Lua. Not only can you write standalone Lua programs, but also integrate Lua code into your OPL programs, making it possible to write applications utilizing Psion's features, such as GUI. Lua is available from FreEPOC.
You can also run Java programs on your Series 5 machine (Psion runs the Java Virtual Machine, programs need to be compiled on a PC). Among other, less known languages, is Prolog and even... a Brainfuck interpreter 🙂
Useful links:
- "Programming Psion Computers" by Leigh Edwards and other free e-books and manuals for both SIBO and EPOC programming
- Programming manuals for Psion Organiser II
- HD6303 and ARM assembly mnemonics are described in the abovementioned packages (Assembler for the Org II) or on their websites (ASM for Psion 5)
- Official home pages, where programming tutorials can be found: Python, Perl, Lua, Java
- [1]Actually, Organiser's OPL is not a good example, since it uses a slightly different syntax (e.g. no PROC-ENDP statements). But programs developed for the Series 3, even those with GUI, should work on Symbian.↩
Programming graphics on Portfolio, part 1
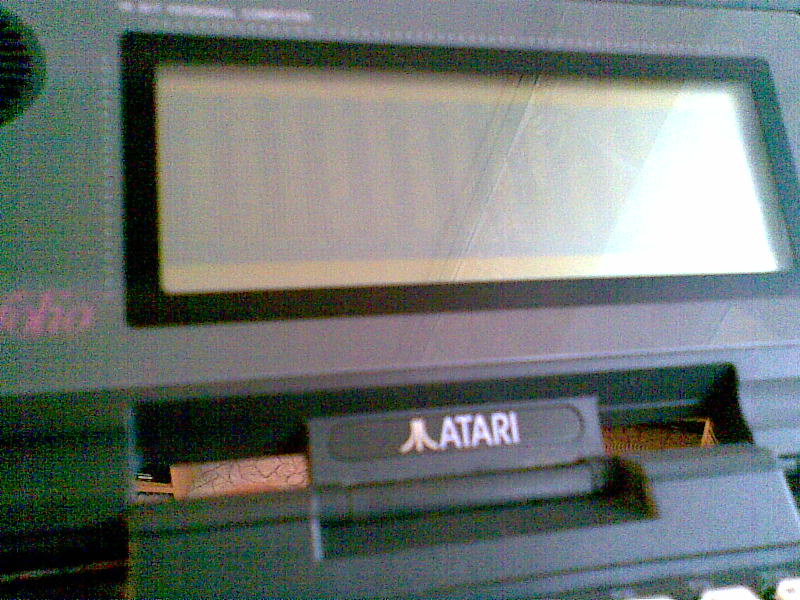
One of Atari Portfolio's weak points is the graphics. Not only has Portfolio very modest graphics capabilites (what can be understood), but also is hardly compatible with PCs. I think this is why there were so few games written for Pofo (Folidash and Phoenix are two of them).
There are two ways to display graphics on Portfolio: using interrupt 10h or sending data directly to the graphics controller. The former is very slow, so it's only intended to use when you want to introduce simple graphic elements (e.g. in board games), but its advantage is the compatibility - you'll be able to run your program on a PC. The latter is much faster - it's actually the only way to program animations - but your code will hang the PC.
Using int 10h is also much easier. If you want to draw an oblique line, you may type:
mov ax, 4 ; set graphics mode 4 int 10h mov cx, 0 ; initial X coordinate mov dx, 0 ; initial Y loop: mov ax,0c01h ; set pixel mov bh, 0 int 10h cmp cx, 63 je end inc cx inc dx jmp loop end: mov ah, 1 ; wait for a keypress int 21h mov ax, 7 ; set graphics mode 7 int 10h int 20h ; exit to DOS