Programming graphics on Portfolio, part 2
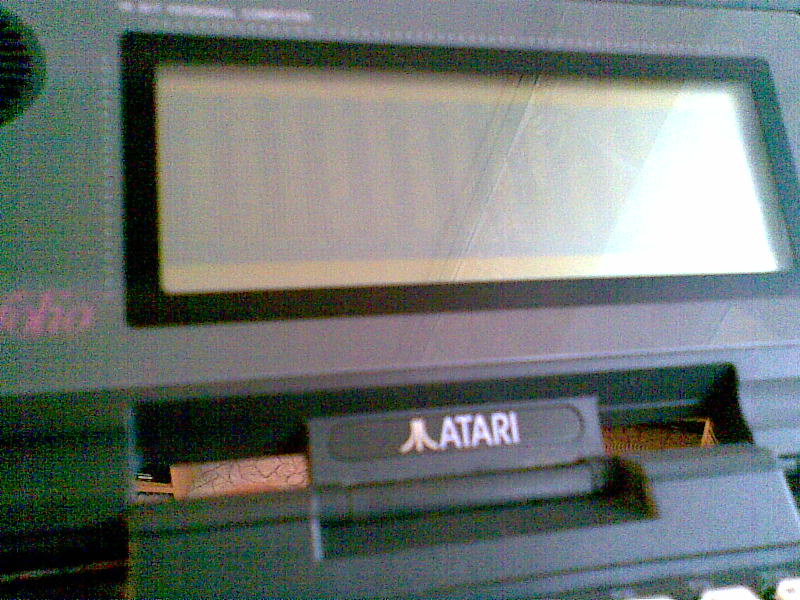
Portfolio's display is controlled by a Hitachi HD61830 chip. As I previously said, this makes it incompatible with the PC and more difficult to program. However, the only way to use dynamic graphics in your programs is to access the controller directly.
If you'd like to update the graphics in real time (e.g. in a game), the best idea would be to redraw the screen at once, so that you don't need to bother drawing each pixel separately. It is possible thanks to the routine by Milan and Martin Hrdlička:
refresh: cld push ax push cx push dx push bx push si push di push ds mov si, 0 mov ax, 0b000h mov ds, ax mov di, 64 ref2: mov cx, 30 mov bx, si mov al, 0ah mov dx, 8011h cli out dx, al mov al, bl dec dx out dx, al sti mov al, 0bh inc dx cli out dx, al dec dx mov al, bh and al, 7 out dx, al sti ref1: lodsb ror al, 1 mov ah, al and ah, 136 ror al, 1 ror al, 1 mov bl, al and bl, 68 or ah, bl ror al, 1 ror al, 1 mov bl, al and bl, 34 or ah, bl ror al, 1 ror al, 1 and al, 17 or al, ah mov ah, al inc dx mov al, 0ch cli out dx, al mov al, ah dec dx out dx, al sti loop ref1 dec di jnz ref2 pop ds pop di pop si pop bx pop dx pop cx pop ax ret
Now, all you need to do is to update the graphics at B000:0000 each time before refreshing the screen. One pixel is one bit, so there are 64 lines by 240/8=30 bytes. That makes 1920 bytes and the actual position of a pixel in memory may be calculated using this formula:
byte = y * 30 + x / 8
bit = x mod 8
so, for example to draw the point at x=50, y=17 (0,0 is the top-left corner, 239,63 is the bottom-right) you need to set the bit number 2 at B000:0204 (that is 516 decimal). Obviously, clearing the bit clears the pixel.
More tips on programming graphics:
- Portfolio News #4
- An article by Martin Řehák (in Czech)
August 10th, 2011 - 23:15
The screen refresh would be faster if you did not mirror every byte of the bitmap. On a PC, the MSB of a byte in the graphics memory corresponds to leftmost pixel of that byte, but it’s the other way round for the HD61830 (see http://leute.server.de/peichl/vidram.gif). IMO there’s no point using the same layout for the Portfolio as is used on a PC – the bitmap is still incompatible due to its dimension of 240×64 pixels.
August 13th, 2011 - 14:50
Thanks for your reply. Sure, the refresh routine would be even faster when we assume, that the bits should be already mirrored in the graphics memory. This, however, forces us to change the formula for x to something like:
bit = 7 - ( x mod 8 )
.January 25th, 2019 - 07:21
Isn’t there a missing push bx in the beginning?
January 26th, 2019 - 12:20
Good point, thanks!